반응형
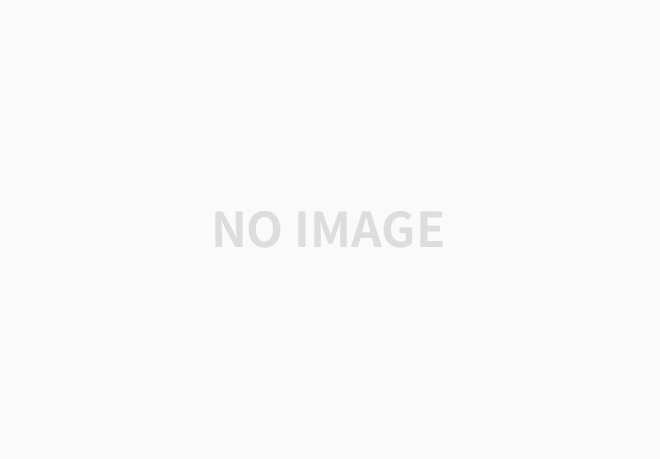
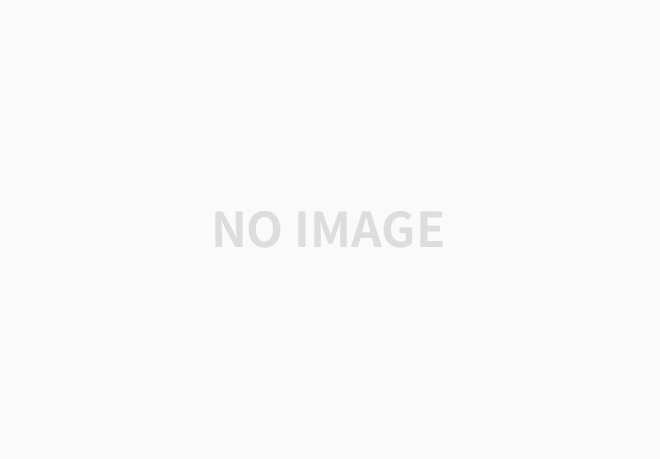
<template>
<div>
<fieldset class="field-container">
<input type="text" placeholder="Search..." class="field" v-model="skillInput" @input="submitAutoComplete" style="margin-bottom : 15px;" />
<div class="icons-container">
<div class="icon-search"></div>
<div class="icon-close">
<div class="x-up"></div>
<div class="x-down"></div>
</div>
</div>
<div class="autocomplete disabled" style="border-radius: 2px;
position: absolute;
top: 100%;
left: 45px;
text-align: left;
font-size: 14px;">
<div @click="searchSkillAdd"
style="cursor: pointer; width: 220px; background: white; padding: 10px 10px;border-bottom: 1px solid #efefef;"
v-for="(res,i) in result"
:key="i" >{{ res }}</div>
</div>
</fieldset>
</div>
</template>
<script>
import skills from "./skills.js";
export default {
data(){
return {
newTodoTtem:"",
skillInput: null,
result: null,
}
},
methods: {
submitAutoComplete() {
const autocomplete = document.querySelector(".autocomplete");
if (this.skillInput) {
autocomplete.classList.remove("disabled");
this.result = skills.filter((skill) => {
return skill.match(new RegExp("^" + this.skillInput, "i"));
});
} else {
autocomplete.classList.add("disabled");
}
},
}
};
</script>
<style lang="scss" scoped>
$hint: #FAF8F8;
$turqoise: #17EAD9;
$cornflower-blue: #6078EA;
$tundora: #4B4848;
$electric-violet: #7C26F8;
$snappy: cubic-bezier(0.694, 0.048, 0.335, 1.000);
@keyframes spin {
0% {
transform: rotate(0deg);
}
100% {
transform: rotate(360deg);
}
}
@keyframes color-1 {
0% {
background-color: #EB73B9;
}
100% {
background-color: #17EAD9;
}
}
::selection {
background: $cornflower-blue;
color: white;
text-shadow: none;
}
::-webkit-selection{
background: $cornflower-blue;
color: white;
text-shadow: none;
}
body {
font-family: 'Montserrat', 'Helevtica', sans-serif;
position: relative;
width: 100%;
height: 100vh;
background-color: darken($hint, 4%);
color: $tundora;
display: flex;
justify-content: center;
align-items: center;
transition: background-color 1.25s ease-in-out;
&.is-focus {
background-color: $turqoise;
}
}
.title-container {
position: absolute;
top: 38%;
left: 50%;
width: 80%;
margin: 0 auto;
text-align: center;
overflow: hidden;
transform: translate(-50%, -50%);
.title {
transform: translateY(-100%);
transition: transform 0.3s ease;
transition-delay: 0.25s;
margin: 0;
color: $cornflower-blue;
}
.title-down {
transform: translateY(100%);
transition: transform 0.3s ease;
transition-delay: 0.25s;
margin: 0;
color: $cornflower-blue;
}
.is-focus & {
.title {
transform: translateY(0);
}
}
.is-type & {
.title-down {
transform: translateY(-30px);
}
.title {
transform: translateY(-100%);
}
}
}
.field-container {
position: relative;
padding: 0;
margin: 0;
border: 0;
width: 330px;
height: 40px;
}
.icons-container {
position: absolute;
top: 0;
right: 52px;
width: 30px;
height: 30px;
overflow: hidden;
}
.icon-close {
position: absolute;
top: 6px;
left: 2px;
width: 75%;
height: 75%;
opacity: 0;
cursor: pointer;
transform: translateX(-200%);
border-radius: 50%;
transition: opacity 0.25s ease, transform 0.43s $snappy;
&:before {
content: "";
border-radius: 50%;
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
opacity: 0;
border: 2px solid transparent;
border-top-color: $cornflower-blue;
border-left-color: $cornflower-blue;
border-bottom-color: $cornflower-blue;
transition: opacity 0.2s ease;
}
.x-up {
position: relative;
width: 100%;
height: 50%;
&:before {
content: "";
position: absolute;
bottom: 1px;
left: 2px;
width: 50%;
height: 2px;
background-color: #51b377;
transform: rotate(45deg);
}
&:after {
content: "";
position: absolute;
bottom: 1px;
right: 0px;
width: 50%;
height: 2px;
background-color: #51b377;
transform: rotate(-45deg);
}
}
.x-down {
position: relative;
width: 100%;
height: 50%;
&:before {
content: "";
position: absolute;
top: 5px;
left: 3px;
width: 50%;
height: 2px;
background-color: #51b377;
transform: rotate(-45deg);
}
&:after {
content: "";
position: absolute;
top: 5px;
right: 1px;
width: 50%;
height: 2px;
background-color: #51b377;
transform: rotate(45deg);
}
}
.is-type & {
&:before {
opacity: 1;
animation: spin 0.85s infinite;
}
.x-up {
&:before, &:after {
animation: color-1 0.85s infinite;
}
&:after {
animation-delay: 0.3s;
}
}
.x-down {
&:before, &:after {
animation: color-1 0.85s infinite;
}
&:before {
animation-delay: 0.2s;
}
&:after {
animation-delay: 0.1s;
}
}
}
}
.icon-search {
position: relative;
top: 7px;
left: 4px;
width: 40%;
height: 40%;
opacity: 1;
border-radius: 50%;
border: 3px solid #51b377;
// border: 3px solid mix($cornflower-blue, white, 35%);
transition: opacity 0.25s ease, transform 0.43s $snappy;
&:after {
content: "";
position: absolute;
bottom: -8px;
right: -4px;
width: 4px;
border-radius: 3px;
transform: rotate(-45deg);
height: 10px;
background-color: #51b377;
// background-color: mix($cornflower-blue, white, 35%);
}
}
.field {
border: 0;
width: 220px;
height: 20px;
padding: 10px 10px;
background: white;
border-radius: 3px;
box-shadow: 0px 8px 15px rgba($tundora, 0.1);
transition: all 0.4s ease;
&:focus {
outline: none;
box-shadow: 0px 9px 20px rgba($tundora, 0.3);
+ .icons-container {
.icon-close {
opacity: 1;
transform: translateX(0);
}
.icon-search {
opacity: 0;
transform: translateX(200%);
}
}
}
}
</style>
반응형
'실무 > vue.js 실무 기능' 카테고리의 다른 글
6. vue.js | @clikc=""시 뒤로가기, 앞으로가기, main 이동 (0) | 2022.10.31 |
---|---|
5. vue.js | SearchBar 자동완성 기능_코드, 클릭시 링크 이동 (0) | 2022.09.29 |
4. vue.js SearchBar 자동완성_참고 포스팅 모음 (0) | 2022.09.29 |
2. setTimeout() 으로 1초후 vue.js transition list기능 (스르륵 나타나게) (0) | 2022.08.18 |
1. setTimeout() 으로 1초후 vue.js transition fade기능 (안보였다가 천천히 보여짐) (0) | 2022.08.16 |